Introduction
Welcome to JsonUI Scripting documentation!
JsonUI Scripting
JsonUI Scripting is an open-source library that uses the JavaScript programming language to build a JsonUI pack with class structures that make it easy to manipulate and edit the UI of Minecraft: Bedrock Edition.
This library also includes a built-in Binding compiler, enabling the use of operators and functions that JsonUI lacks, making binding code more convenient and easier to code!
Installation
To install it into your project, you need to have Node.js pre-installed to use it!
You also need to create a project and use the following command to start using JsonUI Scripting:
Batch
# Use npm npm install jsonui-scripting # Or use yarn yarn add jsonui-scripting
That command will add the JsonUI Scripting library to your project, and you can start using it.
How to use
The syntax is very simple. If you just want to display the text "Hello World" on the main screen, here is the code for that:
Javascript
const { UI, Files, Anchor } = require("jsonui-scripting"); const label = UI.label({ text: "Hello World!", anchor: Anchor.TopMiddle, y: 15, layer: 50, }); Files.StartScreen("start_screen_content").addChild(label);
And you just need to run the code you wrote, and here is the result:
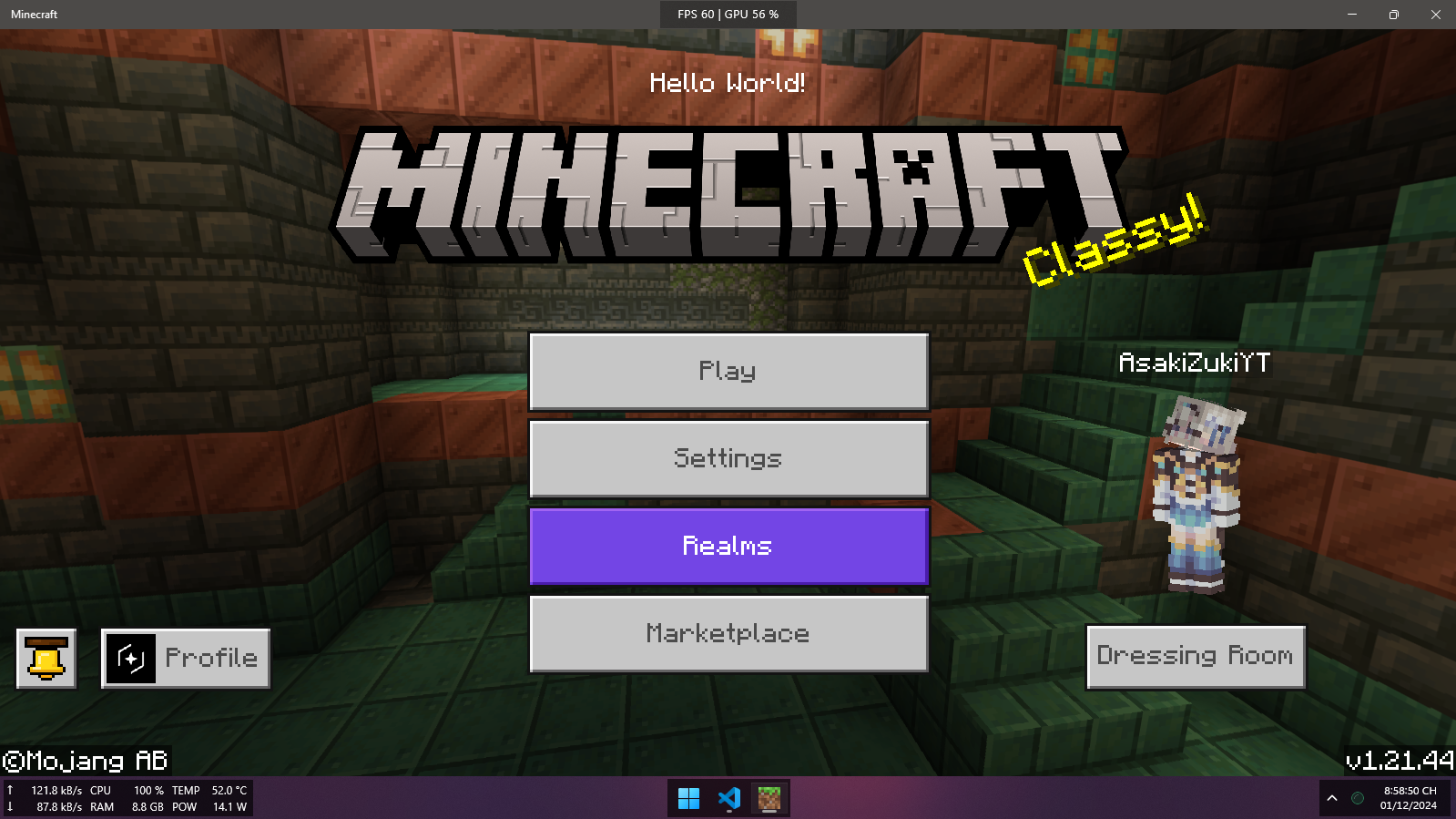